抽屉导航器
抽屉导航器在屏幕一侧渲染一个导航抽屉,可以通过手势打开和关闭。
¥Drawer Navigator renders a navigation drawer on the side of the screen which can be opened and closed via gestures.
这封装了 react-native-drawer-layout
。如果你想在不集成 React Navigation 的情况下使用抽屉,请直接使用该库。
¥This wraps react-native-drawer-layout
. If you want to use the drawer without React Navigation integration, use the library directly instead.
安装
¥Installation
要使用此导航器,请确保你有 @react-navigation/native
及其依赖(遵循本指南),然后安装 @react-navigation/drawer
:
¥To use this navigator, ensure that you have @react-navigation/native
and its dependencies (follow this guide), then install @react-navigation/drawer
:
- npm
- Yarn
- pnpm
npm install @react-navigation/drawer
yarn add @react-navigation/drawer
pnpm add @react-navigation/drawer
然后,你需要安装和配置抽屉导航器所需的库:
¥Then, you need to install and configure the libraries that are required by the drawer navigator:
-
首先,安装
react-native-gesture-handler
和react-native-reanimated
(至少是版本 2 或 3)。¥First, install
react-native-gesture-handler
andreact-native-reanimated
(at least version 2 or 3).如果你有 Expo 托管项目,请在项目目录中运行:
¥If you have a Expo managed project, in your project directory, run:
npx expo install react-native-gesture-handler react-native-reanimated
如果你有一个裸露的 React Native 项目,请在项目目录中运行:
¥If you have a bare React Native project, in your project directory, run:
- npm
- Yarn
- pnpm
npm install react-native-gesture-handler react-native-reanimated
yarn add react-native-gesture-handler react-native-reanimated
pnpm add react-native-gesture-handler react-native-reanimated
-
在 安装指南 之后在项目中配置 Reanimated Babel 插件。
¥Configure the Reanimated Babel Plugin in your project following the installation guide.
-
要完成
react-native-gesture-handler
的安装,我们需要有条件地导入它。为此,请创建 2 个文件:¥To finalize the installation of
react-native-gesture-handler
, we need to conditionally import it. To do this, create 2 files:gesture-handler.native.js// Only import react-native-gesture-handler on native platforms
import 'react-native-gesture-handler';gesture-handler.js// Don't import react-native-gesture-handler on web
现在,在你的入口文件(例如
index.js
或App.js
)的顶部添加以下内容(确保它位于顶部并且之前没有其他内容):¥Now, add the following at the top (make sure it's at the top and there's nothing else before it) of your entry file, such as
index.js
orApp.js
:import './gesture-handler';
由于抽屉导航器在 Web 上不使用
react-native-gesture-handler
,因此可以避免不必要地增加包大小。¥Since the drawer navigator doesn't use
react-native-gesture-handler
on Web, this avoids unnecessarily increasing the bundle size.警告如果你正在为 Android 或 iOS 进行构建,请不要跳过此步骤,否则即使你的应用在开发中运行良好,也可能会在生产中崩溃。这不适用于其他平台。
¥If you are building for Android or iOS, do not skip this step, or your app may crash in production even if it works fine in development. This is not applicable to other platforms.
-
如果你使用的是 Mac 并针对 iOS 进行开发,则还需要安装 pod(通过 可可足类)来完成链接。
¥If you're on a Mac and developing for iOS, you also need to install the pods (via Cocoapods) to complete the linking.
npx pod-install ios
用法
¥Usage
要使用此导航器,请从 @react-navigation/drawer
导入它:
¥To use this navigator, import it from @react-navigation/drawer
:
- Static
- Dynamic
import { createDrawerNavigator } from '@react-navigation/drawer';
const MyDrawer = createDrawerNavigator({
screens: {
Home: HomeScreen,
Profile: ProfileScreen,
},
});
import { createDrawerNavigator } from '@react-navigation/drawer';
const Drawer = createDrawerNavigator();
function MyDrawer() {
return (
<Drawer.Navigator>
<Drawer.Screen name="Home" component={HomeScreen} />
<Drawer.Screen name="Profile" component={ProfileScreen} />
</Drawer.Navigator>
);
}
API 定义
¥API Definition
属性
¥Props
除了所有导航器共享的 常用属性 之外,抽屉导航器组件还接受以下附加属性:
¥In addition to the common props shared by all navigators, the drawer navigator component accepts the following additional props:
backBehavior
这控制在导航器中调用 goBack
时发生的情况。这包括在 Android 上按设备的后退按钮或后退手势。
¥This controls what happens when goBack
is called in the navigator. This includes pressing the device's back button or back gesture on Android.
它支持以下值:
¥It supports the following values:
-
firstRoute
- 返回导航器中定义的第一个屏幕(默认)¥
firstRoute
- return to the first screen defined in the navigator (default) -
initialRoute
- 返回initialRouteName
prop 中传入的初始屏幕,如果不传则默认到第一屏幕¥
initialRoute
- return to initial screen passed ininitialRouteName
prop, if not passed, defaults to the first screen -
order
- 返回到聚焦屏幕之前定义的屏幕¥
order
- return to screen defined before the focused screen -
history
- 返回导航器中上次访问的屏幕;如果多次访问同一屏幕,较旧的条目将从历史记录中删除¥
history
- return to last visited screen in the navigator; if the same screen is visited multiple times, the older entries are dropped from the history -
none
- 不处理后退按钮¥
none
- do not handle back button
defaultStatus
抽屉默认状态 - 抽屉默认是 open
还是 closed
。
¥The default status of the drawer - whether the drawer should stay open
or closed
by default.
当此项设置为 open
时,抽屉将从初始渲染中打开。它可以使用手势或以编程方式正常关闭。然而,当回去时,如果抽屉是关闭的,它会重新打开。这本质上与抽屉的默认行为相反,抽屉从 closed
开始,后退按钮关闭打开的抽屉。
¥When this is set to open
, the drawer will be open from the initial render. It can be closed normally using gestures or programmatically. However, when going back, the drawer will re-open if it was closed. This is essentially the opposite of the default behavior of the drawer where it starts closed
, and the back button closes an open drawer.
detachInactiveScreens
布尔值用于指示是否应从视图层次结构中分离非活动屏幕以节省内存。这使得能够与 react-native-screens 集成。默认为 true
。
¥Boolean used to indicate whether inactive screens should be detached from the view hierarchy to save memory. This enables integration with react-native-screens. Defaults to true
.
drawerContent
返回 React 元素以渲染为抽屉内容的函数,例如导航项
¥Function that returns React element to render as the content of the drawer, for example, navigation items
内容组件默认接收以下属性:
¥The content component receives the following props by default:
-
state
- 导航仪的 导航状态。¥
state
- The navigation state of the navigator. -
navigation
- 导航器的导航对象。¥
navigation
- The navigation object for the navigator. -
descriptors
- 包含抽屉屏幕选项的描述符对象。这些选项可以在descriptors[route.key].options
访问。¥
descriptors
- An descriptor object containing options for the drawer screens. The options can be accessed atdescriptors[route.key].options
.
提供定制 drawerContent
¥Providing a custom drawerContent
抽屉的默认组件是可滚动的,并且仅包含 RouteConfig 中路由的链接。你可以轻松覆盖默认组件,以将页眉、页脚或其他内容添加到抽屉中。默认内容组件导出为 DrawerContent
。它在 ScrollView
内渲染 DrawerItemList
组件。
¥The default component for the drawer is scrollable and only contains links for the routes in the RouteConfig. You can easily override the default component to add a header, footer, or other content to the drawer. The default content component is exported as DrawerContent
. It renders a DrawerItemList
component inside a ScrollView
.
默认情况下,抽屉是可滚动的并支持带有凹口的设备。如果你自定义内容,你可以使用 DrawerContentScrollView
来自动处理:
¥By default, the drawer is scrollable and supports devices with notches. If you customize the content, you can use DrawerContentScrollView
to handle this automatically:
import {
DrawerContentScrollView,
DrawerItemList,
} from '@react-navigation/drawer';
function CustomDrawerContent(props) {
return (
<DrawerContentScrollView {...props}>
<DrawerItemList {...props} />
</DrawerContentScrollView>
);
}
要在抽屉中添加其他项目,你可以使用 DrawerItem
组件:
¥To add additional items in the drawer, you can use the DrawerItem
component:
function CustomDrawerContent(props) {
return (
<DrawerContentScrollView {...props}>
<DrawerItemList {...props} />
<DrawerItem
label="Help"
onPress={() => Linking.openURL('https://mywebsite.com/help')}
/>
</DrawerContentScrollView>
);
}
DrawerItem
组件接受以下属性:
¥The DrawerItem
component accepts the following props:
-
label
(必填):项目的标签文本。可以是字符串,也可以是返回 React 元素的函数。例如({ focused, color }) => <Text style={{ color }}>{focused ? 'Focused text' : 'Unfocused text'}</Text>
。¥
label
(required): The label text of the item. Can be string, or a function returning a react element. e.g.({ focused, color }) => <Text style={{ color }}>{focused ? 'Focused text' : 'Unfocused text'}</Text>
. -
icon
:显示项目的图标。接受返回 React 元素的函数。例如({ focused, color, size }) => <Icon color={color} size={size} name={focused ? 'heart' : 'heart-outline'} />
。¥
icon
: Icon to display for the item. Accepts a function returning a react element. e.g.({ focused, color, size }) => <Icon color={color} size={size} name={focused ? 'heart' : 'heart-outline'} />
. -
focused
:布尔值,指示是否将抽屉项目高亮为活动状态。¥
focused
: Boolean indicating whether to highlight the drawer item as active. -
onPress
(必填):按下时执行的函数。¥
onPress
(required): Function to execute on press. -
activeTintColor
:项目处于活动状态时图标和标签的颜色。¥
activeTintColor
: Color for the icon and label when the item is active. -
inactiveTintColor
:项目处于非活动状态时图标和标签的颜色。¥
inactiveTintColor
: Color for the icon and label when the item is inactive. -
activeBackgroundColor
:项目处于活动状态时的背景颜色。¥
activeBackgroundColor
: Background color for item when it's active. -
inactiveBackgroundColor
:项目处于非活动状态时的背景颜色。¥
inactiveBackgroundColor
: Background color for item when it's inactive. -
labelStyle
:标签Text
的样式对象。¥
labelStyle
: Style object for the labelText
. -
style
:封装器View
的样式对象。¥
style
: Style object for the wrapperView
.
请注意,你不能在 drawerContent
内部使用 useNavigation
钩子,因为 useNavigation
只能在屏幕内部使用。你将获得 drawerContent
的 navigation
属性,你可以使用它来代替:
¥Note that you cannot use the useNavigation
hook inside the drawerContent
since useNavigation
is only available inside screens. You get a navigation
prop for your drawerContent
which you can use instead:
function CustomDrawerContent({ navigation }) {
return (
<Button
onPress={() => {
// Navigate using the `navigation` prop that you received
navigation.navigate('SomeScreen');
}}
>
Go somewhere
</Button>
);
}
要使用自定义组件,我们需要将其传递到 drawerContent
属性中:
¥To use the custom component, we need to pass it in the drawerContent
prop:
<Drawer.Navigator drawerContent={(props) => <CustomDrawerContent {...props} />}>
{/* screens */}
</Drawer.Navigator>
选项
¥Options
以下 options 可用于配置导航器中的屏幕。这些可以在 Drawer.navigator
的 screenOptions
属性或 Drawer.Screen
的 options
属性下指定。
¥The following options can be used to configure the screens in the navigator. These can be specified under screenOptions
prop of Drawer.navigator
or options
prop of Drawer.Screen
.
title
可用作 headerTitle
和 drawerLabel
后备的通用标题。
¥A generic title that can be used as a fallback for headerTitle
and drawerLabel
.
lazy
该屏幕是否应在第一次访问时渲染。默认为 true
。如果你想在初始渲染时渲染屏幕,请将其设置为 false
。
¥Whether this screen should render the first time it's accessed. Defaults to true
. Set it to false
if you want to render the screen on initial render.
drawerLabel
给定 { focused: boolean, color: string }
的字符串或函数返回一个 React.Node,以显示在抽屉侧边栏中。未定义时,使用场景 title
。
¥String or a function that given { focused: boolean, color: string }
returns a React.Node, to display in drawer sidebar. When undefined, scene title
is used.
drawerIcon
函数,给定 { focused: boolean, color: string, size: number }
返回一个 React.Node 以显示在抽屉侧边栏中。
¥Function, that given { focused: boolean, color: string, size: number }
returns a React.Node to display in drawer sidebar.
drawerActiveTintColor
抽屉中活动项目的图标和标签的颜色。
¥Color for the icon and label in the active item in the drawer.
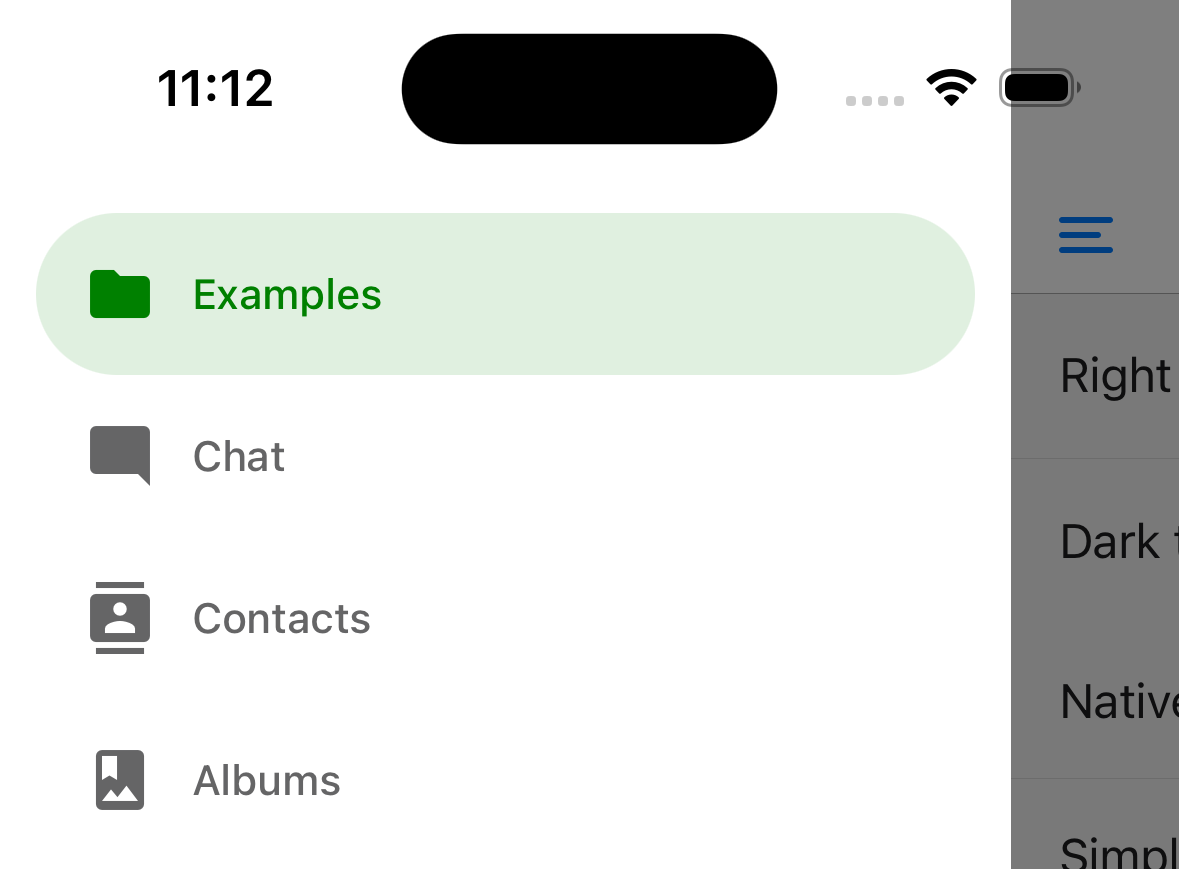
drawerActiveTintColor: 'green',
drawerActiveBackgroundColor
抽屉中活动项目的背景颜色。
¥Background color for the active item in the drawer.
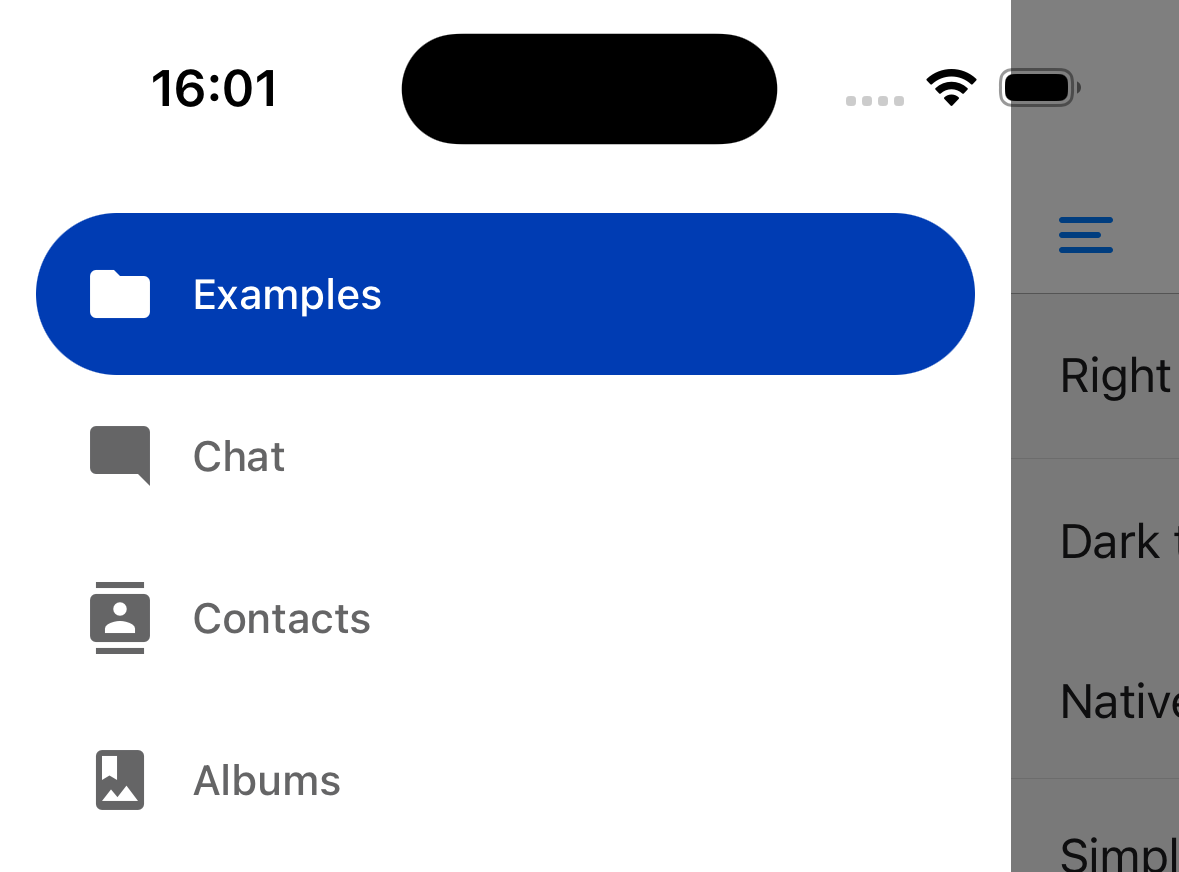
screenOptions={{
drawerActiveTintColor: 'white',
drawerActiveBackgroundColor: '#003CB3',
drawerLabelStyle: {
color: 'white',
},
}}
drawerInactiveTintColor
抽屉中非活动项目的图标和标签的颜色。
¥Color for the icon and label in the inactive items in the drawer.
drawerInactiveBackgroundColor
抽屉中非活动项目的背景颜色。
¥Background color for the inactive items in the drawer.
drawerItemStyle
单个项目的样式对象,可以包含图标和/或标签。
¥Style object for the single item, which can contain an icon and/or a label.
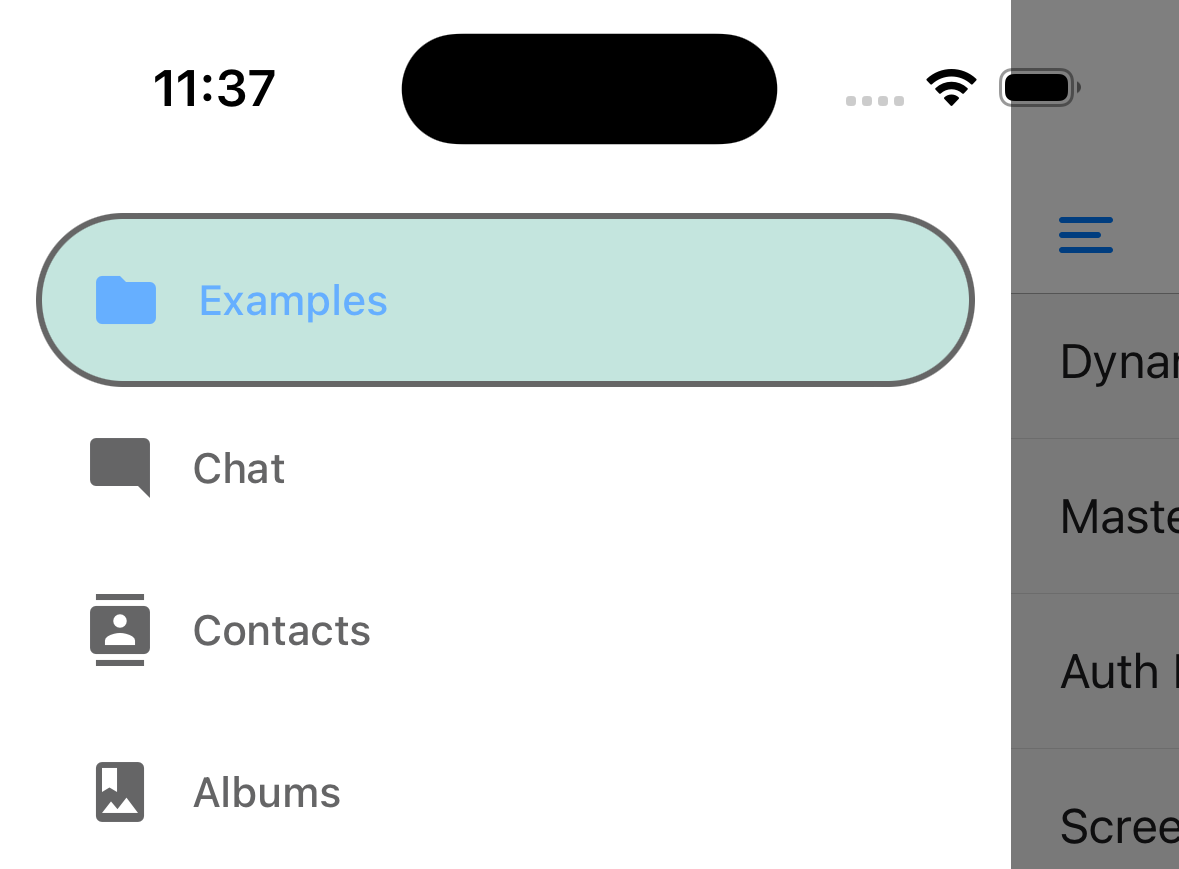
示例:
¥Example:
drawerItemStyle: {
backgroundColor: '#9dd3c8',
borderColor: 'black',
orderWidth: 2,
opacity: 0.6,
},
drawerLabelStyle
应用于渲染标签的内容部分内的 Text
样式的样式对象。
¥Style object to apply to the Text
style inside content section which renders a label.
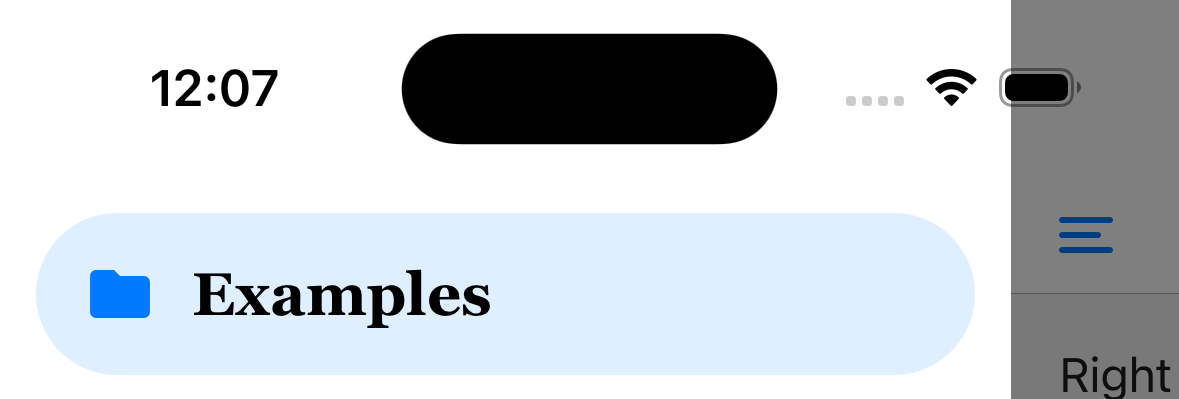
示例:
¥Example:
drawerLabelStyle: {
color: 'black',
fontSize: 20,
fontFamily: 'Georgia',
},
drawerContentContainerStyle
ScrollView
中内容部分的样式对象。
¥Style object for the content section inside the ScrollView
.
drawerContentStyle
封装视图的样式对象。
¥Style object for the wrapper view.
drawerStyle
抽屉组件的样式对象。你可以在此处传递抽屉的自定义背景颜色或自定义宽度。
¥Style object for the drawer component. You can pass a custom background color for a drawer or a custom width here.
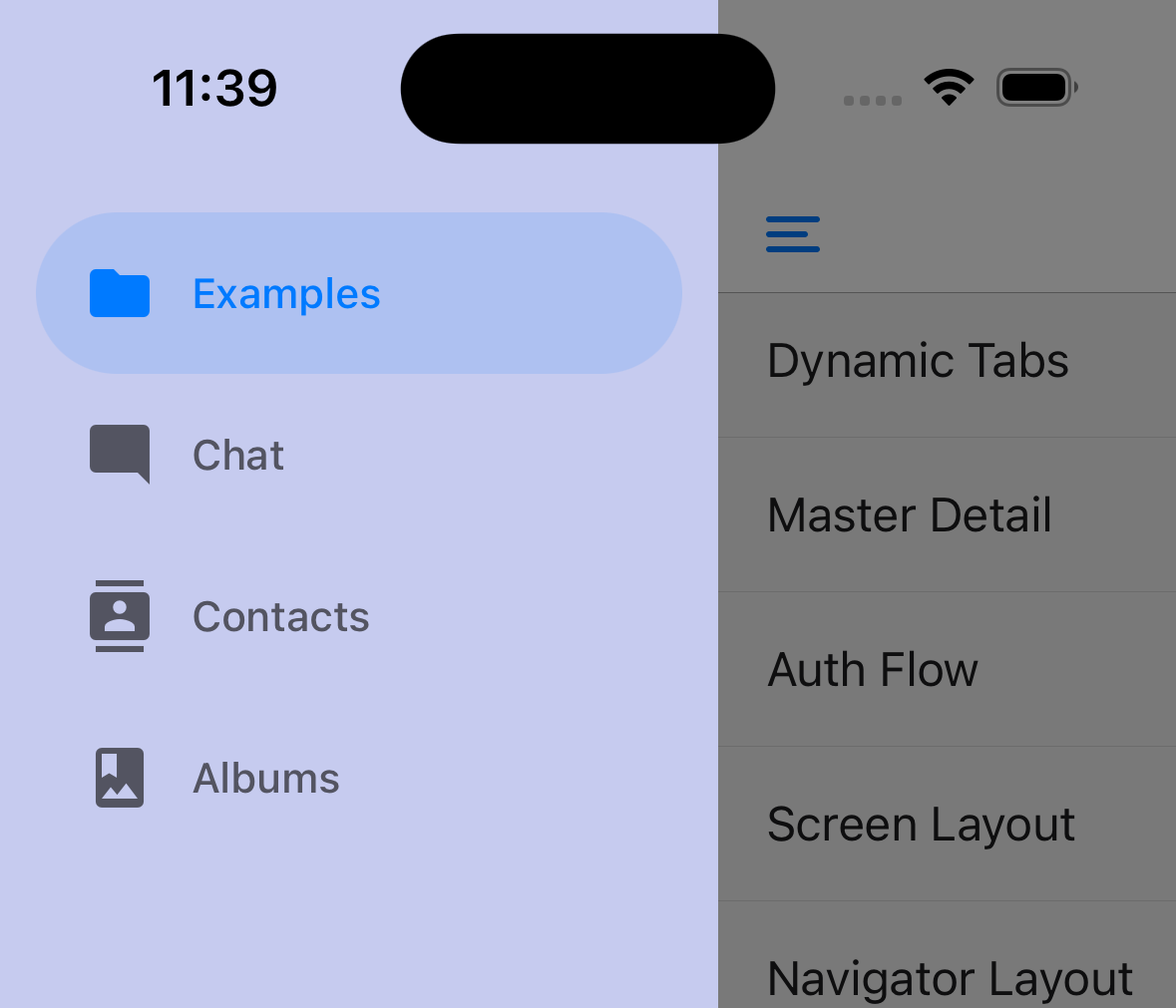
<Drawer.Navigator
screenOptions={{
drawerStyle: {
backgroundColor: '#c6cbef',
width: 240,
},
}}
>
{/* screens */}
</Drawer.Navigator>
drawerPosition
选项为 left
或 right
。LTR 语言默认为 left
,RTL 语言默认为 right
。
¥Options are left
or right
. Defaults to left
for LTR languages and right
for RTL languages.
drawerType
抽屉的类型。它决定抽屉的外观和动画效果。
¥Type of the drawer. It determines how the drawer looks and animates.
-
front
:传统的抽屉,用覆盖层覆盖屏幕。¥
front
: Traditional drawer which covers the screen with an overlay behind it. -
back
:滑动时抽屉会显示在屏幕后面。¥
back
: The drawer is revealed behind the screen on swipe. -
slide
:屏幕和抽屉都会滑动以显示抽屉。¥
slide
: Both the screen and the drawer slide on swipe to reveal the drawer. -
permanent
:永久抽屉显示为侧边栏。对于在大屏幕上始终可见的抽屉很有用。¥
permanent
: A permanent drawer is shown as a sidebar. Useful for having always visible drawer on larger screens.
在 iOS 上默认为 slide
,在其他平台上默认为 front
。
¥Defaults to slide
on iOS and front
on other platforms.
你可以有条件地指定 drawerType
在较大屏幕上显示永久抽屉,在小屏幕上显示传统抽屉抽屉:
¥You can conditionally specify the drawerType
to show a permanent drawer on bigger screens and a traditional drawer drawer on small screens:
import { useWindowDimensions } from 'react-native';
import { createDrawerNavigator } from '@react-navigation/drawer';
const Drawer = createDrawerNavigator();
function MyDrawer() {
const dimensions = useWindowDimensions();
return (
<Drawer.Navigator
screenOptions={{
drawerType: dimensions.width >= 768 ? 'permanent' : 'front',
}}
>
{/* Screens */}
</Drawer.Navigator>
);
}
你还可以根据屏幕尺寸指定其他属性(例如 drawerStyle
)来自定义行为。例如,你可以将其与 defaultStatus="open"
结合起来实现主从布局:
¥You can also specify other props such as drawerStyle
based on screen size to customize the behavior. For example, you can combine it with defaultStatus="open"
to achieve a master-detail layout:
import { useWindowDimensions } from 'react-native';
import { createDrawerNavigator } from '@react-navigation/drawer';
const Drawer = createDrawerNavigator();
function MyDrawer() {
const dimensions = useWindowDimensions();
const isLargeScreen = dimensions.width >= 768;
return (
<Drawer.Navigator
defaultStatus="open"
screenOptions={{
drawerType: isLargeScreen ? 'permanent' : 'back',
drawerStyle: isLargeScreen ? null : { width: '100%' },
overlayColor: 'transparent',
}}
>
{/* Screens */}
</Drawer.Navigator>
);
}
drawerHideStatusBarOnOpen
当设置为 true
时,只要抽屉被拉出或处于 "open" 状态,抽屉就会隐藏操作系统状态栏。
¥When set to true
, Drawer will hide the OS status bar whenever the drawer is pulled or when it's in an "open" state.
drawerStatusBarAnimation
隐藏状态栏时的动画。与 drawerHideStatusBarOnOpen
结合使用。
¥Animation of the statusbar when hiding it. use in combination with drawerHideStatusBarOnOpen
.
仅 iOS 支持此功能。默认为 slide
。
¥This is only supported on iOS. Defaults to slide
.
支持的值:
¥Supported values:
-
slide
-
fade
-
none
overlayColor
当抽屉打开时,颜色叠加显示在内容视图的顶部。当抽屉打开时,不透明度会从 0
动画到 1
。
¥Color overlay to be displayed on top of the content view when drawer gets open. The opacity is animated from 0
to 1
when the drawer opens.
sceneContainerStyle
封装屏幕内容的组件的样式对象。
¥Style object for the component wrapping the screen content.
gestureHandlerProps
传递给底层平移手势处理程序的属性。
¥Props to pass to the underlying pan gesture handler.
Web 上不支持此功能。
¥This is not supported on Web.
swipeEnabled
是否可以使用滑动手势打开或关闭抽屉。默认为 true
。
¥Whether you can use swipe gestures to open or close the drawer. Defaults to true
.
Web 上不支持滑动手势。
¥Swipe gesture is not supported on Web.
swipeEdgeWidth
允许定义滑动手势应激活距内容视图边缘多远。
¥Allows for defining how far from the edge of the content view the swipe gesture should activate.
Web 上不支持此功能。
¥This is not supported on Web.
swipeMinDistance
应激活打开抽屉的最小滑动距离阈值。
¥Minimum swipe distance threshold that should activate opening the drawer.
keyboardDismissMode
当滑动手势开始时是否应关闭键盘。默认为 'on-drag'
。设置为 'none'
以禁用键盘处理。
¥Whether the keyboard should be dismissed when the swipe gesture begins. Defaults to 'on-drag'
. Set to 'none'
to disable keyboard handling.
freezeOnBlur
布尔值,指示是否阻止非活动屏幕重新渲染。默认为 false
。当 react-native-screens
包中的 enableFreeze()
在应用顶部运行时,默认为 true
。
¥Boolean indicating whether to prevent inactive screens from re-rendering. Defaults to false
.
Defaults to true
when enableFreeze()
from react-native-screens
package is run at the top of the application.
仅支持 iOS 和 Android。
¥Only supported on iOS and Android.
popToTopOnBlur
布尔值,表示在离开此抽屉屏幕时是否应将任何嵌套堆栈弹出到堆栈顶部。默认为 false
。
¥Boolean indicating whether any nested stack should be popped to the top of the stack when navigating away from this drawer screen. Defaults to false
.
它仅在抽屉导航器下嵌套有堆栈导航器(例如 堆栈导航器 或 原生堆栈导航器)时才有效。
¥It only works when there is a stack navigator (e.g. stack navigator or native stack navigator) nested under the drawer navigator.
标题相关选项
¥Header related options
你可以找到标题相关选项 此处 的列表。这些 options 可以在 Drawer.navigator
的 screenOptions
属性或 Drawer.Screen
的 options
属性下指定。你不必直接使用 @react-navigation/elements
来使用这些选项,它们只是记录在该页面中。
¥You can find the list of header related options here. These options can be specified under screenOptions
prop of Drawer.navigator
or options
prop of Drawer.Screen
. You don't have to be using @react-navigation/elements
directly to use these options, they are just documented in that page.
除此之外,抽屉中还支持以下选项:
¥In addition to those, the following options are also supported in drawer:
header
使用自定义标头代替默认标头。
¥Custom header to use instead of the default header.
它接受一个返回 React 元素以显示为标题的函数。该函数接收一个包含以下属性的对象作为参数:
¥This accepts a function that returns a React Element to display as a header. The function receives an object containing the following properties as the argument:
-
navigation
- 当前屏幕的导航对象。¥
navigation
- The navigation object for the current screen. -
route
- 当前屏幕的路由对象。¥
route
- The route object for the current screen. -
options
- 当前屏幕的选项¥
options
- The options for the current screen -
layout
- 屏幕尺寸,包含height
和width
属性。¥
layout
- Dimensions of the screen, containsheight
andwidth
properties.
示例:
¥Example:
import { getHeaderTitle } from '@react-navigation/elements';
// ..
header: ({ navigation, route, options }) => {
const title = getHeaderTitle(options, route.name);
return <MyHeader title={title} style={options.headerStyle} />;
};
要为导航器中的所有屏幕设置自定义标题,你可以在导航器的 screenOptions
属性中指定此选项。
¥To set a custom header for all the screens in the navigator, you can specify this option in the screenOptions
prop of the navigator.
在 headerStyle
中指定 height
¥Specify a height
in headerStyle
如果你的自定义标题高度与默认标题高度不同,那么你可能会注意到由于测量异步而导致的故障。明确指定高度将避免此类故障。
¥If your custom header's height differs from the default header height, then you might notice glitches due to measurement being async. Explicitly specifying the height will avoid such glitches.
示例:
¥Example:
headerStyle: {
height: 80, // Specify the height of your custom header
};
请注意,默认情况下,此样式不会应用于标头,因为你可以控制自定义标头的样式。如果你也想将此样式应用于标题,请使用 props 中的 options.headerStyle
。
¥Note that this style is not applied to the header by default since you control the styling of your custom header. If you also want to apply this style to your header, use options.headerStyle
from the props.
headerShown
是否显示或隐藏屏幕标题。默认情况下显示标题。将其设置为 false
会隐藏标题。
¥Whether to show or hide the header for the screen. The header is shown by default. Setting this to false
hides the header.
活动
¥Events
导航器可以对某些操作进行 触发事件。支持的事件有:
¥The navigator can emit events on certain actions. Supported events are:
drawerItemPress
当用户按下抽屉中屏幕的按钮时会触发此事件。默认情况下,抽屉式物品压机会执行以下几项操作:
¥This event is fired when the user presses the button for the screen in the drawer. By default a drawer item press does several things:
-
如果屏幕未聚焦,按下抽屉项目将使该屏幕聚焦
¥If the screen is not focused, drawer item press will focus that screen
-
如果屏幕已经聚焦,那么它将关闭抽屉
¥If the screen is already focused, then it'll close the drawer
要防止默认行为,你可以调用 event.preventDefault
:
¥To prevent the default behavior, you can call event.preventDefault
:
React.useEffect(() => {
const unsubscribe = navigation.addListener('drawerItemPress', (e) => {
// Prevent default behavior
e.preventDefault();
// Do something manually
// ...
});
return unsubscribe;
}, [navigation]);
如果你有自定义抽屉内容,请确保发出此事件。
¥If you have custom drawer content, make sure to emit this event.
帮手
¥Helpers
抽屉导航器向导航对象添加以下方法:
¥The drawer navigator adds the following methods to the navigation object:
openDrawer
打开抽屉面板。
¥Opens the drawer pane.
navigation.openDrawer();
closeDrawer
关闭抽屉面板。
¥Closes the drawer pane.
navigation.closeDrawer();
toggleDrawer
如果抽屉窗格已关闭,则打开抽屉窗格;如果抽屉窗格已打开,则将其关闭。
¥Opens the drawer pane if closed, closes the drawer pane if opened.
navigation.toggleDrawer();
jumpTo
导航至抽屉式导航器中的现有屏幕。该方法接受以下参数:
¥Navigates to an existing screen in the drawer navigator. The method accepts the following arguments:
-
name
- string - 要跳转到的路由名称。¥
name
- string - Name of the route to jump to. -
params
- object - 屏幕参数传递到目标路由。¥
params
- object - Screen params to pass to the destination route.
navigation.jumpTo('Profile', { owner: 'Satya' });
钩子
¥Hooks
抽屉导航器导出以下钩子:
¥The drawer navigator exports the following hooks:
useDrawerProgress
此钩子返回抽屉的进度。它在抽屉导航器渲染的屏幕组件以及 自定义抽屉内容 中都可用。
¥This hook returns the progress of the drawer. It is available in the screen components rendered by the drawer navigator as well as in the custom drawer content.
progress
对象是一个 SharedValue
,它表示抽屉的动画位置(0
已关闭;1
已打开)。它可用于根据 复活 的抽屉动画为元素设置动画:
¥The progress
object is a SharedValue
that represents the animated position of the drawer (0
is closed; 1
is open). It can be used to animate elements based on the animation of the drawer with Reanimated:
- Static
- Dynamic
import {
createDrawerNavigator,
useDrawerProgress,
} from '@react-navigation/drawer';
import Animated, { useAnimatedStyle } from 'react-native-reanimated';
function HomeScreen() {
const progress = useDrawerProgress();
const animatedStyle = useAnimatedStyle(() => ({
transform: [{ translateX: progress.value * -100 }],
}));
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Animated.View
style={[
{
height: 100,
aspectRatio: 1,
backgroundColor: 'tomato',
},
animatedStyle,
]}
/>
</View>
);
}
import {
createDrawerNavigator,
useDrawerProgress,
} from '@react-navigation/drawer';
import Animated, { useAnimatedStyle } from 'react-native-reanimated';
function HomeScreen() {
const progress = useDrawerProgress();
const animatedStyle = useAnimatedStyle(() => ({
transform: [{ translateX: progress.value * -100 }],
}));
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Animated.View
style={[
{
height: 100,
aspectRatio: 1,
backgroundColor: 'tomato',
},
animatedStyle,
]}
/>
</View>
);
}
如果你使用类组件,则可以使用 DrawerProgressContext
来获取进度值。
¥If you are using class components, you can use the DrawerProgressContext
to get the progress value.
useDrawerProgress
钩子(或 DrawerProgressContext
)将在 Web 上返回一个模拟值,因为 Reanimated 未在 Web 上使用。模拟值只能表示抽屉的打开状态(关闭时为 0
,打开时为 1
),而不能表示抽屉的进度。
¥The useDrawerProgress
hook (or DrawerProgressContext
) will return a mock value on Web since Reanimated is not used on Web. The mock value can only represent the open state of the drawer (0
when closed, 1
when open), and not the progress of the drawer.
useDrawerStatus
你可以使用 useDrawerStatus
钩子检查抽屉是否打开。
¥You can check if the drawer is open by using the useDrawerStatus
hook.
import { useDrawerStatus } from '@react-navigation/drawer';
// ...
const isDrawerOpen = useDrawerStatus() === 'open';
如果你不能使用钩子,你也可以使用 getDrawerStatusFromState
助手:
¥If you can't use the hook, you can also use the getDrawerStatusFromState
helper:
import { getDrawerStatusFromState } from '@react-navigation/drawer';
// ...
const isDrawerOpen = getDrawerStatusFromState(navigation.getState()) === 'open';
对于类组件,你可以监听 state
事件来检查抽屉是打开还是关闭:
¥For class components, you can listen to the state
event to check if the drawer was opened or closed:
class Profile extends React.Component {
componentDidMount() {
this._unsubscribe = navigation.addListener('state', () => {
const isDrawerOpen =
getDrawerStatusFromState(navigation.getState()) === 'open';
// do something
});
}
componentWillUnmount() {
this._unsubscribe();
}
render() {
// Content of the component
}
}
将抽屉导航器嵌套在其他导航器中
¥Nesting drawer navigators inside others
如果抽屉式导航器嵌套在提供某些 UI 的另一个导航器(例如选项卡导航器或堆栈导航器)内,则抽屉将在这些导航器的 UI 下方渲染。抽屉将出现在选项卡栏下方和堆栈标题下方。你需要将抽屉式导航器设为任何导航器的父级,其中抽屉式导航器应渲染在其 UI 之上。
¥If a drawer navigator is nested inside of another navigator that provides some UI, for example, a tab navigator or stack navigator, then the drawer will be rendered below the UI from those navigators. The drawer will appear below the tab bar and below the header of the stack. You will need to make the drawer navigator the parent of any navigator where the drawer should be rendered on top of its UI.